The data layer is an array object on JavaScript-enabled front ends. It is used to hold intelligence data in JSON format on the client side so that third-party tools can consume it and read it on the server side.
“Intelligence data” are advertising, marketing, and/or contextual data and metadata that relates to the past and present and describes the website, its content, the things it relates to, who the users are, and how they interact with it.
These data can include, but are not limited to, information about:
- The website
- Website ID
- Website title
- Website domain name
- Website country
- Website language
- The page
- Page ID
- Page title
- Page path
- Page content
- The user
- User ID
- Hashed PII
- Hashed email address
- Hashed phone number
- The engagement
- Hit or view
- Horizontal or vertical scroll
- Click
- Share
- Listen
- Watch
- Play
- Close or quit
- The transactions
- Cart
- Add to cart
- Remove from cart
- Purchase
- Purchase ID
- Campaign and/or coupon
- Product
- Product ID and/or SKU
- Product name
- Product price
- Cart
- The experience
- Performance
- Errors
Simply put, a data layer is a log of what this page is about—and how the user interacted with it. This log contains the single version of the truth, and is referenced by all server-side tools that need to collect data from the client side.
A data layer is also a bridge between frontend and backend, and vise versa. By pushing and pulling data to and from it, different components of your website or web application exchange information with one another in the same format.
Why Implement a Data Layer
From a technical perspective, there are numerous benefits to implementing a data layer on your website or web application. Arguably, the two biggest benefits are that with a data layer, you can:
- Apply the architectural principles of having a Single Source of Truth (SSOT) and Single Version of the Truth (SVOT) for intelligence data and metadata.
- Standardize this intelligence data and metadata in JSON format, so that it becomes accessible and usable for all third-party tools that need to consume and ingest it.
- Reduce the time and resources needed to collect data from frontends across third-party tools by pushing to a single and centralized place once, then referring to it if (and as) needed.
In the rest of this article, we’ll explore how to create and use the data layer.
How to Implement a Data Layer
In its simplest form, a data layer implementation consists of three steps. You need to create a data layer, push data elements to it (from upstream; the frontend), and then pull data elements from it (to downstream; the backend).
Creating the Data Layer
How to declare a data layer:
To create a data layer, declare an empty array object with the name you want:
// Declare dataLayer array object
window.dataLayer = window.dataLayer || [];
How the object is named—and how to set the data elements that you push to it—comes down to the context and requirements of your implementation.
(Some sites refer to “data elements” and others to “data items” being pushed to the data layer. For the purposes of this article, a data element and data item mean the same thing: itelligence information held in one index of the array object.)
As a general rule of thumb, websites that use Google Tag Manager and Google Analytics have a dataLayer
object, whereas those that use Adobe Launch and Adobe Analytics have a digitalData
object.
Where to put your data layer declaration:
The data layer array object is declared at (or near) the top of the <head>
tag:
<!doctype html>
<html class="no-js" lang="en">
<head>
<title>Data Layer Demo</title>
<!-- Declare data layer -->
<script>
window.dataLayer = window.dataLayer || [];
</script>
<!-- Embed scripts that push/pull to/from the data layer -->
<script>
dataLayer.push({'key': 'value'});
</script>
<!-- Load scripts that push/pull to/from the data layer -->
<script src="site.com/js/script.js"></script>
</head>
<body>
</body>
</html>
The declaration of the data layer array object must, without exceptions, precede all scripts that need to push data to it or pull data from it.
Otherwise, those scripts will try to reference an object that hasn’t been defined yet, and you will observe a “ReferenceError: dataLayer is not defined” error in the console:
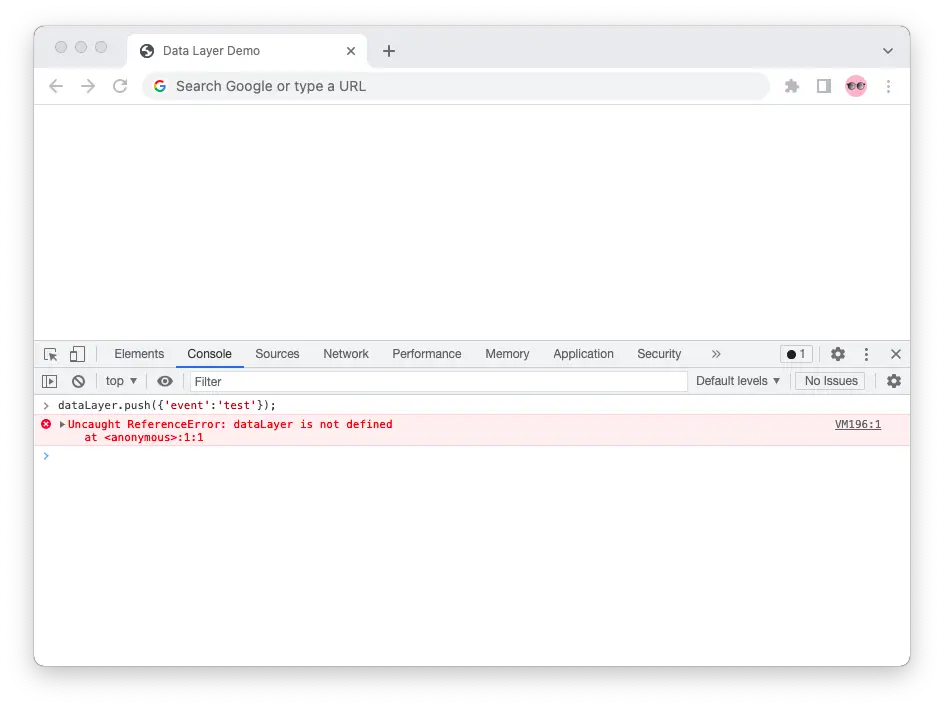
Pushing Data to the Data Layer
To push data to the data layer, you use the Array.push()
method in JavaScript:
// Push one data element with one key/value pair
dataLayer.push({'key': 'value'});
// Push one data element with multiple key/value pairs
dataLayer.push({'key1': 'value1', 'key2': 'value2'});
// Push two data elements
dataLayer.push({'key1': 'value1'}, {'key2': 'value2'});
The dataLayer.push()
method allows you to push one or more data elements to the data layer. Each data element can contain one or more key/value pairs.
Adjacent key/value pairs should be separated by comma. Each data element should be enclosed in curly brackets ({}), and adjacent data elements must be separated by comma.
On success, the elements are added to the end of the array object in the order with which they were pushed. Then, the method returns the array’s new length.
Pushing flat data elements to the data layer:
You can push flat data elements to the data layer, each containing one or more key/value pairs.
For example, the following data layer push:
// Push a flat user event with login state and last login date
dataLayer.push({'event': 'user', 'isLoggedIn': true, 'lastLogin': '1641049200'});
Populates the data layer array object in the following way:
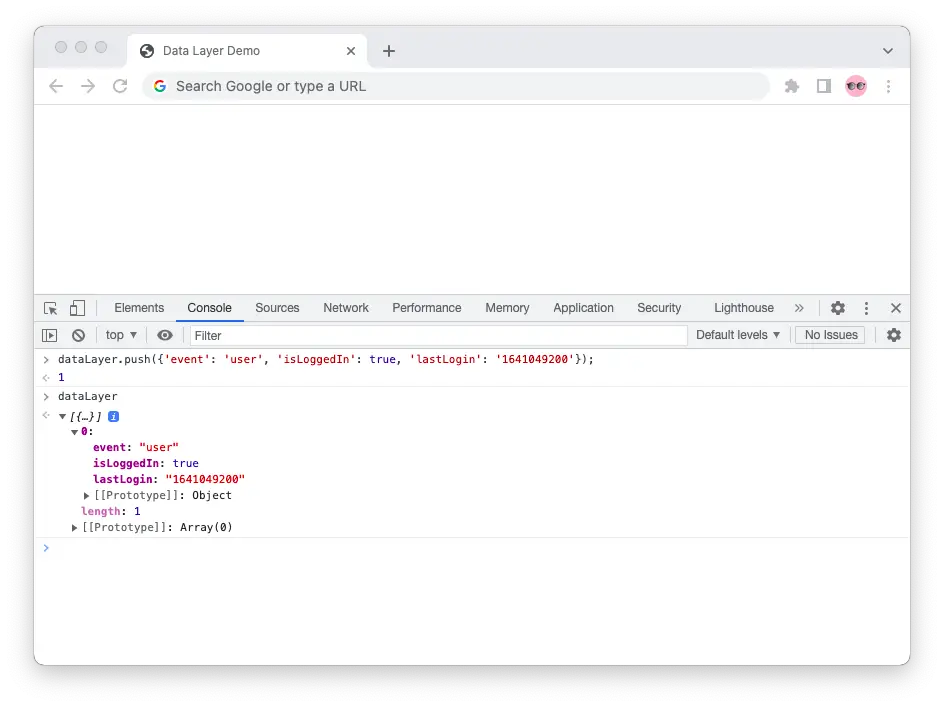
This is the basic and most common implementation of a data layer. The flatness of the data elements pushed makes the data layer easy to debug and pull from.
However, this is less the case the more key/value pairs are pushed per data element. The more key/value pairs per data element needed, the more nesting (see below) starts to make sense.
Pushing nested data elements to the data layer:
You can also push nested data elements to the data layer.
To nest a data element within another data element, creating a parent/child relationship between them, enclose the child data element in curly braces and set it as the value of the parent element’s key/value pair.
For example, if we were to reformat the flat data layer push from the example above to a nested data layer push, our statement would look like this:
// Push a nested user event with login state and last login date
dataLayer.push({
'user': {
'isLoggedIn': true,
'lastLogin': '1641049200'
}
});
And it would populate the data layer array object in the following way:
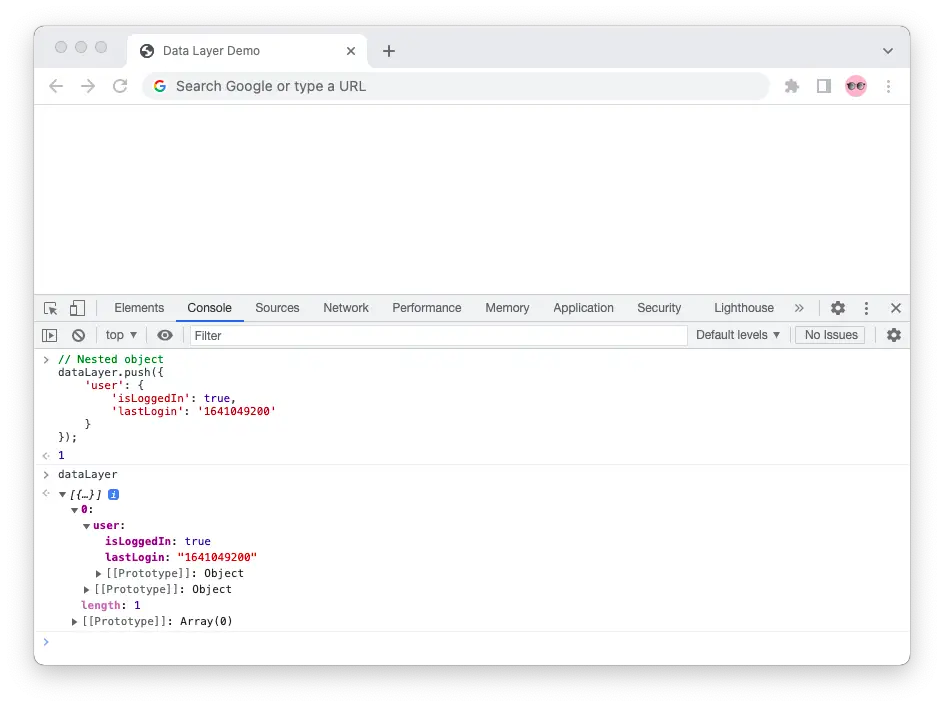
Nested data elements add structure. But, with that structure, they also add complexity, which makes them harder to debug and use, especially for junior developers and beginner users of tag management tools; use them purposefully.
Pulling Data From the Data Layer
Nine times out of ten, you’re likely to use a tag manager, such as Adobe Launch, Google Tag Manager, or Tealium iQ, to extra data elements from the data layer, then transform and load it into downstream destinations, such as ad platforms or analytics tools.
If you need to write code to access the data elements held in the data layer, you simply need to reference the name of the data layer array object.
This, for a data layer named dataLayer
, returns the whole object:
// Return the whole array
dataLayer
And this returns the nth index:
// Return 0th index
dataLayer[0]
// Returns 1st index
dataLayer[1]
// Returns 2nd index
dataLayer[2]
Data Layer: Things to Know
Declare a data layer object early on:
Always make sure that a data layer array object is declared before attempting to push data elements to it. This means that, in the HTML markup, the script with the declaration of the empty data layer object should precede all third-party tools that need to use it, from tag managers to consent managers.
Don’t reassign your data layer object later on:
Never reassign a data layer object. By doing so, you will erase all previously pushed data elements to it, potentially disturbing the logic of your website’s or web application’s data flow on the client side. A data layer is sacred; once declared, it can be pushed to and pulled from—but should never be emptied or overridden.
Remember that the data layer isn’t persistent:
The data layer isn’t persistent. It stores the data you’ve assigned to it until the user closes the page, refreshes the page, or opens another page on the same tab. If you need persistent storage, consider first-party cookies, sessionStorage, localStorage, IndexedDB, or WebSQL instead.
On having multiple data layers:
You can have more than one data layers on the same page (though many would dispute the benefits of doing so). However, they need to be named differently. For example, if your first data layer is named dataLayerA
, the second would need to be named dataLayerB
.