An identifier is a reversed word that tells the JavaScript interpreter that the code about to follow is for a variable, a function, or a property of an object.
For example, when you declare a constant in your code, the identifier is the keyword const
, followed by that constant’s name and the value or object to be stored in it:
const answerToLife = 42;
The keywords var
, let
, class
, and function
are also identifiers.
Identifiers in JavaScript are case-sensitive. As explained in the MDN Web Docs, they can contain Unicode letters, $, _, and digits (0-9), but they must not start with a digit.
This brings us to the dreaded “Uncaught SyntaxError: Unexpected identifier” error.
In JavaScript, the error “Uncaught SyntaxError: Unexpected identifier” occurs when you misspell an identifier for a constant, variable, function, or property of an object.
Usually, this means one of two things:
- You forgot you had “Caps Lock” on, so you typed
Var
instead ofvar
orFUNCTION
instead offunction
- You mistyped the identifier itself, like
fnction
instead offunction
A few examples of misspelled identifiers in your code:
// Incorrect
Var myVar = "A wrongly capitalized variable."
// Correct
var myVar = "A properly declared variable."
// Incorrect
lt anotherVar = "A misspelled let variable."
// Correct
let anotherVar = "A properly declared let variable."
// Incorrect
Function myFunction() { console.log("A wrongly capitalized function."); }
// Correct
function myFunction() { console.log("A wrongly capitalized function."); }
See how the syntax highlighter uses a different color when the identifier is incorrectly capitalized or misspelled? This is your first clue when debugging your code.
Your second clue is the contents of the error message in your browser’s developer console:
To fix the unexpected identifier error, take a good hard look at what the virtual machine statement says to the right of the error message. The number after the colon gives you the line in your code at which the error occurred:
As you can see in the screenshot above, the “Uncaught SyntaxError: Unexpected identifier” occurred in line 3 of my code.
Clicking on it will take you directly to the line of your code that caused the error, and the syntax highlighter will show you the problematic declaration:
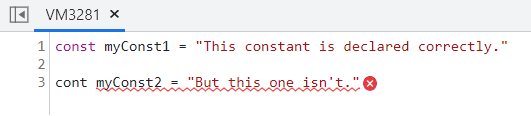
Note that the highlighter has underlined the declaration, not the misspelled identifier. Pay attention to this when debugging your code, or you might go off chasing red herrings.