Suppose that, for one reason or another, you want to repeat a string an n number of times in JavaScript.
What’s the best way to do that?
If you’re in the market for advice, I’d argue it’s by using the repeat()
method. And, in this post, I will share my reasoning as to why.
The repeat()
method in JavaScript takes the string you pass it as a parameter, and then constructs a new string by concatenating the original string as many times as you’ve instructed it to.
It’s as simple and straightforward as JavaScript methods get. And, nine times out of ten, it does the job perfectly.
How It Works
String.prototype.repeat(count)
The repeat()
method expects an integer with a non-negative value from 0 to +Infinity. A few things to keep in mind as you use it:
- If you give it a negative count, it will return a RangeError;
- If you give it a count of 0, it will return an empty string (“”);
- If you give it a decimal count (for example, 2.4), it will convert it to an integer (for example, 2) and won’t truncate the string.
If legacy browser support is important, check out browser compatibility for the repeat()
method and consider using the following polyfill.
An Example
To see how the repeat() method in JavaScript works in practice, let’s construct the refrain from Chumbawamba’s Tubthumping, a rock song everyone who lived through the 1990s will remember.
The refrain goes like this:
I get knocked down, but I get up again
You are never gonna keep me down
I get knocked down, but I get up again
You are never gonna keep me down
I get knocked down, but I get up again
You are never gonna keep me down
I get knocked down, but I get up again
You are never gonna keep me down
Chumbawamba — Tubthumping
In other words, what we have to do is take the first two lines from the song’s refrain, store them in a variable, then pass it onto the repeat()
method with the instruction to repeat it 4 times:
let refrain = "I get knocked down, but I get up again\nYou are never gonna keep me down\n";
console.log(refrain.repeat(4));
To give this function a spin right now and see how the repeat()
method works first-hand, open up your browser’s console and copy/paste the above.
This is what you’re going to see:
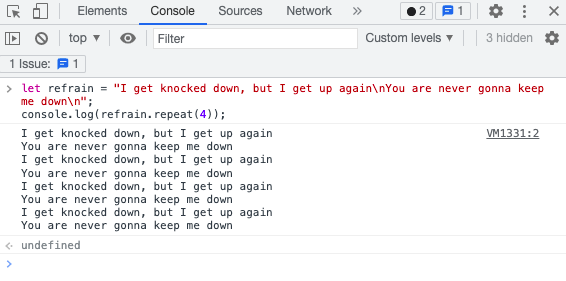
repeat()
method in actionConclusion
This is it! Although there’s more than one way to multiply a string in JavaScript, this is the most direct and by far the easiest.
As with any approach, there are drawback and edge cases. If you can think of any—or came across some in your implementation—be sure to share them with the rest of this post’s readers in the comments section below.