All links in HTML have a single, solid underline. This has been the case since 1993, when version 0.13 of the Mosaic browser came out and introduced underlining of the anchor text for hyperlinks.
For example, the simple HTML markup below:
<!doctype html>
<html class="no-js" lang="en">
<head>
<meta charset="utf-8">
<title>Sample Document</title>
</head>
<body>
<p>This is a sample document with <a href="#">a hyperlink</a>.</p>
</body>
Looks like this when opened in a browser:
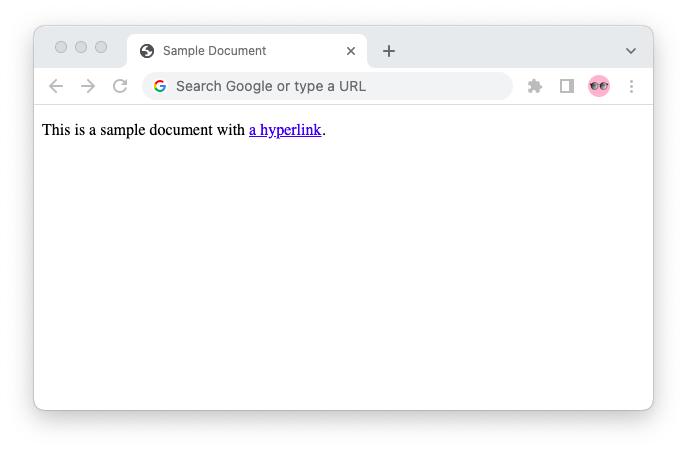
This raises the question: If you don’t want links to be underlined on your website, how can you remove that underlining?
To remove the underline from one or multiple links in your HTML document, give them the CSS property of text-decoration: none
.
This tutorial will teach you exactly how to do this for all links, a group of links, or a single link.
Links in HTML Documents (And Why It Matters)
Browsers apply a default CSS stylesheet to the DOM elements in your HTML documents. For example, these are the default CSS stylesheets of Gecko (Firefox) and of WebKit (Chrome, Safari, others) browsers.
To override this default styling, simply insert one or more CSS stylesheets with your own styles into your HTML document. (If you’re new to HTML/CSS and don’t know how to do this, read on; I’ve explained it later in this post).
In HTML, you create hyperlinks by wrapping anchor text in an <a> tag with a href
attribute.
Links can be “just links,” as shown in the markup below:
<!doctype html>
<html class="no-js" lang="en">
<head>
<meta charset="utf-8">
<title>Sample Document</title>
</head>
<body>
<p>This is <a href="https://google.com/">a link to Google</a>.</p>
</body>
In practice, however, links are usually embedded in navigation menus or within sections, articles, and sidebars:
<!doctype html>
<html class="no-js" lang="en">
<head>
<meta charset="utf-8">
<title>Sample Document</title>
</head>
<body>
<!-- Navigation -->
<nav>
<ul>
<li><a class="your-class" id="your-id-01" href="index.html">Home</a></li>
<li><a class="your-class" id="your-id-02" href="about.html">About</a></li>
<li><a class="your-class" id="your-id-03" href="contacts.html">Contacts</a></li>
</ul>
</nav>
<!-- Article -->
<article>
<p>This is <a href="https://google.com/">a link to Google</a>.</p>
</article>
</body>
</html>
Sometimes, they will have Classes and Ids, such as the links in our <nav>
above. Othertimes, they won’t, such as the link to Google in the <article>
section above.
This is important to know because, as we will discuss in a moment, you can target all links, groups of links, or individual links using CSS selectors depending on what you want to do.
Removing the Underline From Links in HTML
With CSS, you can remove the underline from all links, or only remove the underline from links based on their state, class, or id. This is done using the text-decoration
CSS rule.
From All Links
To remove the underline from all links, regardless of their state, class, or id, add the following rule to your CSS stylesheet:
a, a:hover, a:active {
text-decoration: none;
}
To remove the underline from links based on their state, use the following CSS selectors:
/* Unvisited links */
a:link {
text-decoration: none;
}
/* Visited links */
a:visited {
text-decoration: none;
}
/* Hovered links */
a:hover {
text-decoration: underline;
}
/* Clicked links */
a:active {
text-decoration: none;
}
The example above removes underlines from unvisited, visited, and active links, but keeps it for hovered links.
From Links With a Specific Class
To remove the underline from links based on their class, add the following rule to your CSS stylesheet:
/* Links with .your-class only */
a.your-class, a.your-class:hover, a.your-class:active {
text-decoration: none;
}
Note: Replace your-class
with the name of the CSS class you want to target.
The example above removes underlines for all links with a CSS class of .your-class
while keeping them for the rest.
To remove the underline from links based on their class and state, add the following rule to your CSS stylesheet:
/* Unvisited .your-class links */
a.your-class:link {
text-decoration: none;
}
/* Visited .your-class links */
a.your-class:visited {
text-decoration: none;
}
/* Hovered .your-class links */
a.your-class:hover {
text-decoration: underline;
}
/* Clicked .your-class links */
a.your-class:active {
text-decoration: none;
}
Note: Replace your-class
with the name of the CSS class you want to target.
The example above goes a notch deeper.
It removes underlines from all links with a CSS class of .your-name
but the links with a state of :hover
.
From a Link With a Unique Id
In HTML/CSS, a Class is a group identifier that you can give to many elements, whereas an Id is a unique identifier that you should assign only to one element.
To remove the underline from a specific link with a unique id, add the following rule to your CSS stylesheet:
/* Link with #your-id-01 only */
a#your-id-01, a#your-id-01:hover, a#your-id-01:active {
text-decoration: none;
}
Note: Replace your-id-01
with the name of the CSS identifier you want to target.
To remove the underline from a specific link with a unique id based on its state, add the following rule to your CSS stylesheet:
/* Unvisited .your-class links */
a#your-id-01:link {
text-decoration: none;
}
/* Visited .your-class links */
a#your-id-01:visited {
text-decoration: none;
}
/* Hovered .your-class links */
a#your-id-01:hover {
text-decoration: underline;
}
/* Clicked .your-class links */
a#your-id-01:active {
text-decoration: none;
}
Note: Replace your-id-01
with the name of the CSS identifier you want to target.
The above code removes the underline from the link with an Id of #your-id-01
but keeps it on hover.
In Conclusion
Now you know how to remove underlines from links in your HTML document using CSS. You also know how to apply basic CSS selectors to target links based on their state, class, or id.
If you’re new to front-end development, I highly recommend taking the time to master CSS selectors. Once you learn the ropes, the only limits to styling your HTML documents will be your imagination and ingenuity.