The Selenium IDE is a great tool if you want to create test cases directly in your browser, without the need to write a single line of code in the Selenium WebDriver.
It gives you a simple UI that you can use to record, edit, and run test projects. However, it also takes a little getting used to if you’re new to the tool and you have something specific that you want to do.
Suppose you’re creating a test in the Selenium IDE. Within that test, you want Selenium to iterate over a list and do the same steps for each element in that list. You do this by using the forEach
command to control the flow of your test.
The tutorial below will show you how.
Loop Through the Elements on a List With Selenium
Let’s imagine that we want to create a grocery list for buying apples, bananas, and oranges. Then, we want to echo every food item on that list.
Here’s what the control flow of our Selenium test would look like:
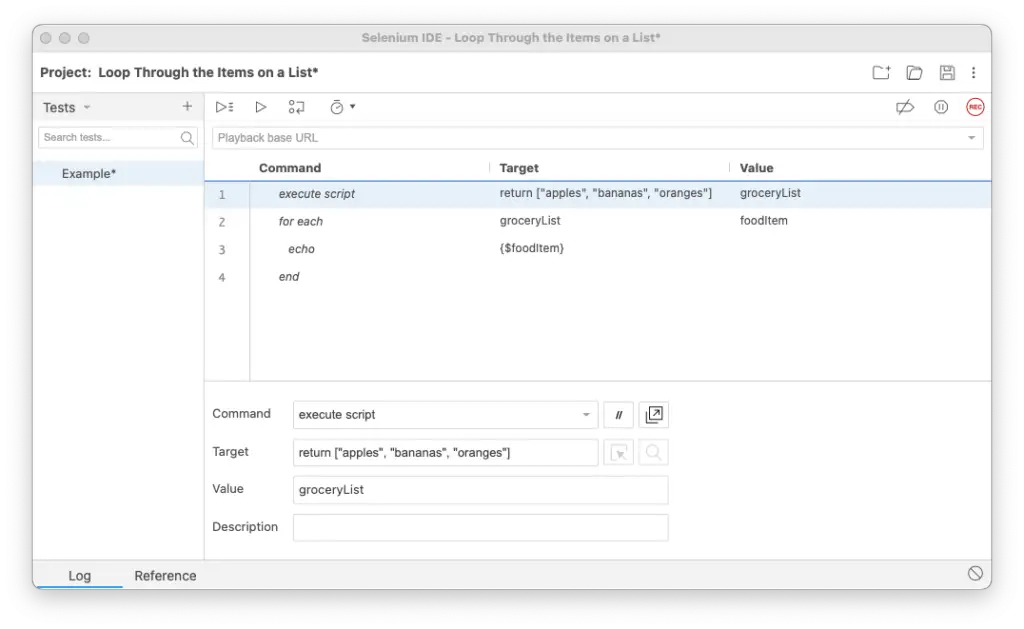
So, what’s happening here?
First, we’re using the execute script
command to run a JavaScript code snippet that returns an Array
object called groceryList
. Apples, bananas, and oranges are stored as elements in that Array
object in indexes 0, 1, and 2, respectively:
// The target of our execute script command
return ["apples", "bananas", "oranges"]
Then, we’re using the forEach
command to iterate over the food items in our shopping list. The name of the food item being looped over is stored in the iterator variable called foodItem
in the “Value” field for the forEach
command.
Finally, we’re echoing the value of the foodItem variable by referencing {$foodItem}
in the “Target” field of an echo
command.
Don’t forget the end
command — without it, Selenium will throw you an error that you’re trying to execute an incomplete block.
Now that you know how to use the forEach
command in Selenium, let’s see what this would look like in a more realistic implementation scenario!
Suppose this is a test case for a grocery shopping web app, and you want to test the ability to add food items to the grocery shopping list through the web app’s UI. Here’s what what the control flow for the Selenium IDE test case could look like:
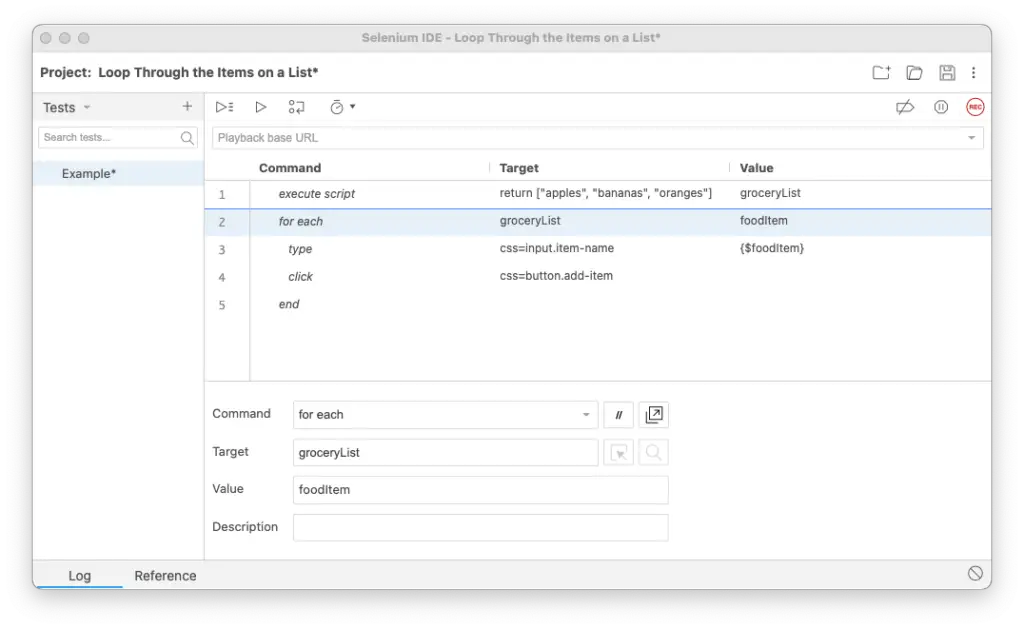
See what we did?
Once again, we created an Array
of food items called groceryList
. We’ve hardcoded the food items, but those might as well come from a different JavaScript code snippet that creates a list of elements by parsing the DOM, for example.
And once again, we looped through the food items in that array and stored their name in the form of a string in the foodItem
iterator variable.
But, this time, we’re targeting the <input>
DOM element with a class name of “item-name” and typing in the value of the food item iterator variable inside it.
Then, we’re adding that food item to the list by clicking the <button>
DOM element with a class name of “add-item”.
In Summary
To loop over a list of items in Selenium IDE, execute a script to store the items in an Array
object and use the forEach
command on that Array
object’s content, storing the value for the current item in an iterator variable.
You can then reference that value in your test case as you need to and where you need to, either as in the “Target” or in the “Value” fields of your commands. And don’t forget to add the end
command to signify the end of your loop!